TDDD49 Programming C# and .NET
C# .Net Lab series 2012
- Recommended deadline 1. Task 1-2. November 23.
- Hard deadline. Final course deadline. All tasks. December: 14. All deliverables should be uploaded on this date.
- Possible re-exam: Next available time for finishing the course: April 10 and August 22 2013.
The labs are centered around WPF and C# coding and use art creation as an example. At the end of the course we will create an art exhibition online and also print some pictures for hanging around IDA. Hopefully we will also be able to formulate an exhibition around art and code at Linköpings Länsmuseeum in the spring 2013. this will of course require your consent.
Interested students looking for a challenge can look at integrating a physics engine into the process for Lab 2 and 3. One example system well suited for this is using the Nine Engine on codeplex (http://nine.codeplex.com/), which is an XNA Game engine integrating physics and also use WPF (or actually XAML) to great extent. If you are interested, willing and able feel free and encouraged to add a system like this after having evaluated it – but it is not required and not supported by the course staff.
Lab 1: Creating a house in WPF
The first lab is focused on WPF binding engine, that automates relations between WPF components and C#-code, other WPF components and persistent data formats such as databases and XML documents.
The task: make a house drawing using WPF shapes using the following components:
- A house body,
- a roof,
- a chimney,
- a door and,
- a window.
These objects must be positioned in a certain way in relation to one another. Implement your version of the following rule set:
- The roof should sit on-top of the house body,
- the chimney should sit on the roof,
- the door should align with the bottom of the house body,
- the window should be on one half of the house body and the door on the other (horizontally) and,
- the window should be positioned in the upper half of the house body (vertically).
Using the binding engine in WPF we are able to create relations between for instance properties in C# classes and WPF components, but also among WPF components.
Implement the following:
- Make the color of the house switch as we hover over it with the mouse.
- Make the house body adapt to a property in a C# class, for instance a random increment. (You may make the property change using a WPF button in the UI to simplify verification, trigger a change in the C# property using somthing like
button1_Click...
.) - Make the roof change its width as the house body changes it width.
- Make the door adapt to the size of the house body. Make your own simple function for this.
- Make the window adapt to the size of the door in WPF directly, without any connection to C# code.
- If you like, experiment to make a nice house...
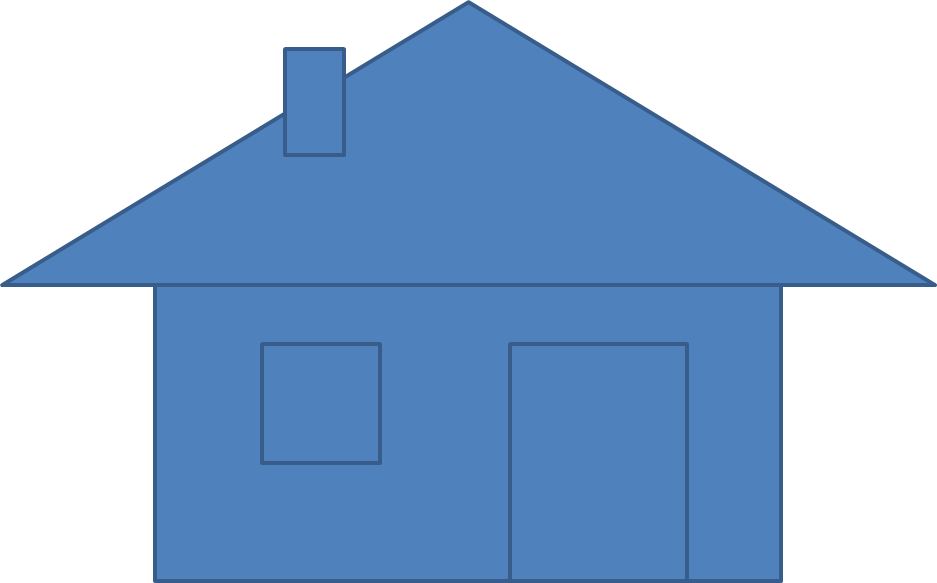
Deliverable: Upload your Visual Studio solution on the TDDD49 forum.
Lab 2: Art tool - city painter
Having learnt a little about the WPF bindings and shapes in WPF, let’s move on to the creation of art tools using a WPF and C# coding. The lab uses a city painting example. But I’d love to see variations on the theme such as a cornfield or a children’s playground or a sky with dragons breathing fire.
For GUI style we want the full screen style with movable dialogs like in Photoshop, MyPaint, or GIMP.
The program should implement the following requirements:- Create a full screen application and handle switching form full screen to windowed mode using Esc.
- Add a color-picker component that enable the user to make a choice of at least 3 specific colors: this could be the sky-color, the house color, and the ground color.
- Here it is ok to use an externa extra color-picker component from WPF or limit the amount of colors avaliabel but the user should be able to choose from some colors.
- Use the selected colors to also generate at least 3 other colors to use for instance by blending the colors such as the sky color and house body color. You can use these colors to define fill colors or stroke-colors for the edges of the shapes, or for other uses. This is up to you but you should use at least 6 colors in total.
- Users should be able to add house bodies with windows and control the placement of both the house body and the windows in the houses. These shapes are interrelated, so the window should not be possible to drag outside of the house body when you add the ability to move the windows and the house.
- The coordinates of windows must be related or somehow bound to the coordinates of the house.
- Add the ability to add, edit and delete houses body and windows.
- Add doors in a similar fashion.
- Enable the addition of a moon or sun to the scene.
- Enable the addition of clouds in multiple layers, where depth is handled by a suitable blending or manipulation of the colors, or by the use of shadows – a darker color = a cloud being further away from the viewer.
- Add functionality to save to PNG or in another format.
- Add the ability to save ongoing work using serialization in a way consitent with the limitations of saving XAML you can find here: http://msdn.microsoft.com/en-us/library/ms754193(v=vs.100).aspx.
- Add the ability to move objects around. There should be conditions defining how things are able to move: such as houses touching the ground and the sun and moons staying in the back, and clouds being in front of the sun or moon, the spacing of windows. (Here you should feel free to implement more rules or the right rules to make the paintings fun and easy to create).
Deliverable: Upload your Visual Studio solution on the TDDD49 forum.
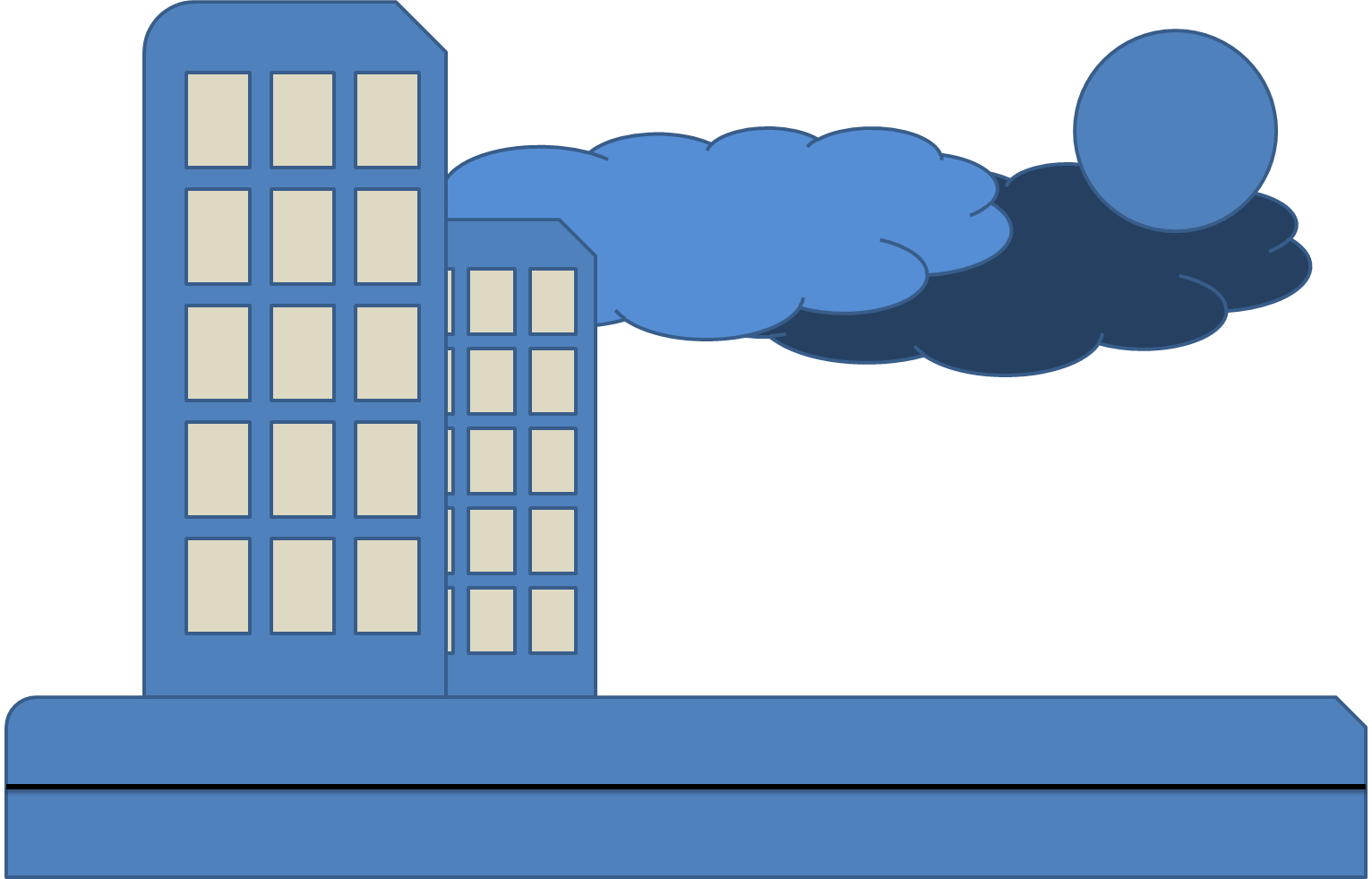
Lab 3: Procedural art generation – example flower bouquet
In this lab we will focus more on algorithmic C# coding based art generation. You can choose to start over or to continue the work started in Lab 2. But the focus here is much more on the volume creation of art using c# classes and methods. Very large amount of details is the goal for the creation of a picture.
To make this more apparent we will look at another example as illustration, the generation of a bouquet of abstract flowers. (It is ok to continue with the city or some other example). Another alternative would simply be to generate a form of abstract art that uses basic shapes like circles and rectangles but builds them up in complex, volumes and patterns with colors blending and so forth.
- A flower is made up of a stem, leaves, flowers, thorns or small extremities.
- For a flower to grow there is the need to make an organic shape that ends with a flower, has some leaves and starts with a relatively clear end stem.
- A bouquet is made up of several flowers joined together to form a kind of an hourglass shape, potentially with the bottom half being much less wide
- The colors of the flowers need to complement each other in two to four colors
- The colors of the stems may vary in two colors
- Some stems will not have leaves or flowers
- Requirement (doing more is definitively ok):
- You procedural art generation tool should use 3-4 different components, such as: stem, leaves, flowers, and thorns
- The placement and amount of the different components should be based on a distribution profile such as (a) 30% of the stems should lack thorns and leaves, (b) 30% of the flowers should have exactly same color. You need to have at least 10 such rules for your program.
- A variation of about 10-20 different colors should be used from a a color base of 3-4 base colors used to generate these variations, using the blending of colors, filters and complementary colors.
- The system should generate a large volume of shapes, think in the region of 1000:s.
- The production of the art should include random elements, but also be based on conditional continuation; the continued generation of shapes should be based on the previously generated shapes. Map this to the distribution profile mentioned earlier and by utilizing this extensively you can ensure variation.
- Allow the user to define base shapes: such as leafs and stem components. The user should define at least two. By defining is meant, for instance, to desfin the points a shapoe is based on using the mouse.
- Save the user-defined shapes for reuse.
- Use the user-defined shapes as a stand point and manipulate them with small changes using the shapes' underlying points and moving them small increments to create variation.
- Add the ability to save pictures and settings for another run.
Deliverable: Screen-cast presentation (see Screen casting software) of the system running and your Visual Studio solution uploaded.

Page responsible: Sahand Sadjadee
Last updated: 2013-01-11