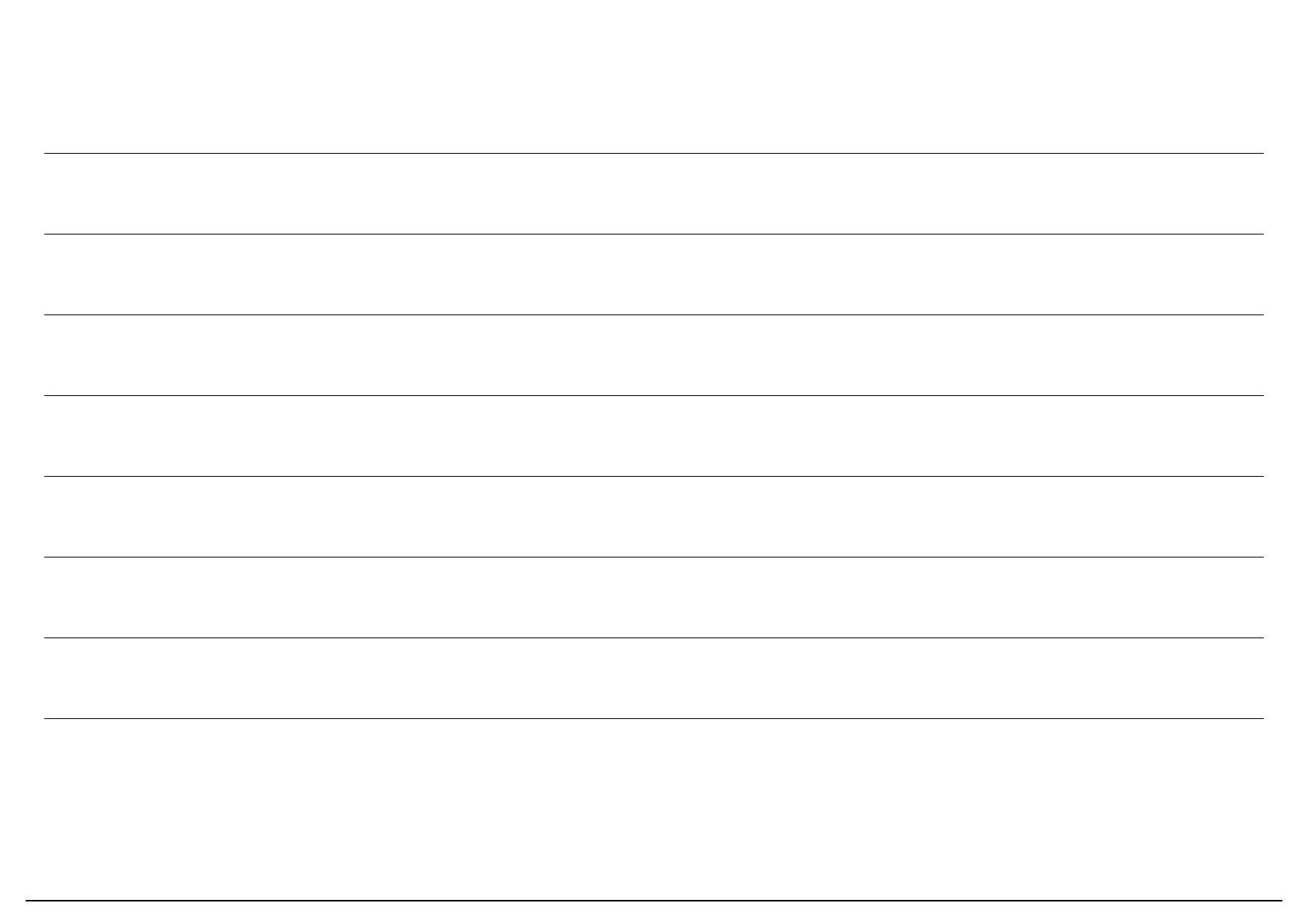
File: Standard-Algorithms-OH-en © 2015 Tommy Olsson, IDA, Linköpings universitet
TDDD38 APiC++ Standard Library – Algorithms 278
Mutating sequence operations (2)
replace(first,last,old,new) substitutes each element in [first,last) equal to old with new
replace_if(first,last,pred,new) substitutes each element in [first,last) for which pred is true with new
iter replace_copy(first,last,result,old,new)asreplace, but the result is copied into another range [result, …)
iter replace_copy_if(first,last,result,pred,new)asreplace_if, but the result is copied into another range [result, …)
iter remove(first,last,value) eliminates all elements in [first,last) equal to value
iter remove_if(first,last,pred) eliminates all elements in [first,last) for which pred is true
iter remove_copy(first,last,result,value)asremove but the result is copied into [result, …)
iter remove_copy_if(first,last,result,pred)asremove_if but the result is copied into [result, …)
iter unique(first,last) eliminates all but the first from every consecutive group of equal elements
iter unique_copy(first,last,result)asunique but the result is copied into [result, …)
reverse(first,last) reverses the order of the elements in [first,last)
iter reverse_copy(first,last,result)asreverse but the result is copied into [result, …)
rotate(first,new-first,last) left rotate the elements in [first,last) so new-first becomes the first element
iter rotate_copy(first,new-first,last,result)asrotate but the result is copied into [result, …)
Note 1:iter returned by the functions above, is an iterator pointing past-the-end of the resulting range.
Note 2: the input range for unique and unique_copy is normally supposed to be sorted.
Note 3: unique and unique_copy also comes in a version taking a predicate, to be used instead of operator== to compare for equality.